Building a liteAPI app on Node.js
Welcome to the liteAPI Node.js cookbook! This guide will help you set up the example Express app on a Node.js server that can run locally. This example app includes searching hotel rates by city, verifying details during a prebook step, accepting payment, and finally booking a room. This is a basic app example meant to show how the core sdk features can be used, so it doesn't incorporate a lot of frills.
Prerequisites
Before you begin, ensure you have the following installed:
Step 1: Clone the Express App Repository
First, clone the provided Express app repository to your local machine: [TK - Repo needs to go live and have key removed and regened]
git clone https://github.com/liteapi-travel/build-website-example
cd build-website-example
Step 2: Install Project Dependencies and Start the App
Navigate to the project directory and install the required dependencies:
npm install
Step 3: Configure Environment Variables
Create a .env
file in the root of your project. The easiest way to do this is to make a copy of the .env.example file and save it as .env
. Add the following environment variables:
PROD_API_KEY=prod_9xxxxxxxxxx #Copy your prod key here
SAND_API_KEY=sand_3dxxxxxxxxx #Copy your sandbox key here
Set your SAND_API_KEY
to your liteAPI sandbox API key. You can use the app without setting the production key. If you have enabled production mode in the dashboard, you can set the PROD_API_KEY
to your production API key. This will allow real bookings to be made through the app. These API keys are how you authenticate to the lite API.
Step 4: Explore the Project Structure
Take a moment to familiarize yourself with the project structure:
project-directory/
├── .env.example
├── .env //That you created in the step above
├── .gitignore
├── README.md
├── package.json
├── package-lock.json
├── node_modules/
├── server/
│ └── server.js
└── client/
├── styles.css
├── index.html
└── app.js
In the client
directory there is a landing page with a form to query rates, index.html
. The index file includes the app.js
script and is styled by style.css
. The app pings the server.js
file to handle API requests.
Step 5: Running the Example App
Start the app with the following command:
npm start
Open your browser and navigate to http://localhost:3000
to see the app in action.
Step 6: Finding Rooms
To search for rooms, start at http://localhost:3000/
which loads the client/index.html
file. Use the default or enter values for city name, country code, check in, check out, and number of guests. You can select the environment key you want to use here. By default you will use the Sandbox API key, where the entire booking flow can be tested, including payment, but won't actually reserve the room or cause any money to be transacted.
If you switch to Production, then a real booking will occur that will charge the payment method used and will generate commission for the production key used.
Hitting the Search Hotels button fires off the searchHotels()
function. This calls the http://localhost:3000/search-hotels
endpoint in the server.js
file.
Endpoint Code:
// Route to search for hotels
app.get("/search-hotels", async (req, res) => {
const { checkin, checkout, adults, city, countryCode } = req.query;
try {
const response = await sdk.getHotels(countryCode, city);
const data = await response;
const first50Ids = data.data.slice(0, 5).map((hotel) => hotel.id);
const result = await sdk.getFullRates(
checkin,
checkout,
"USD",
"US",
first50Ids,
adults
);
res.json({ hotels: result }); // Send a JSON response with the hotels data
} catch (error) {
console.error("Error searching for hotels:", error);
res.status(500).json({ error: "Internal server error" });
}
});
Notice two key endpoint calls happen here. const response = await sdk.getHotels(countryCode, city);
this first call uses the sdk to call getHotels()
passing in the country and city the user entered to get a list of hotel IDs. These hotel IDs are then passed into the sdk's getFullRates()
function along with the other fields. getFullRates()
will return the list of rates in JSON format.
Step 7: Selecting a room
The returned rates are listed on the page and Book Now option will lock in that rate. The next step is entering in the users information, followed by a payment form.
If you are in sandbox mode, use the test card 4242 4242 4242 4242
with any future expiration date and any 3 digits for the security code.
Example:
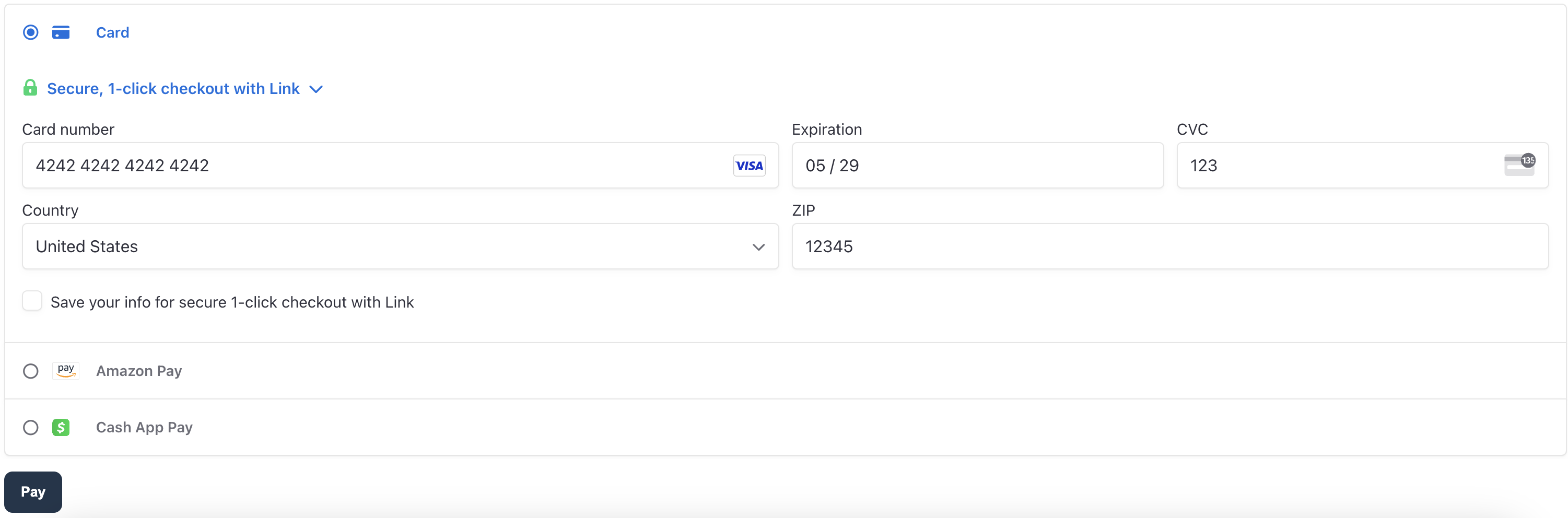
Step 8: Booking the Room
Once the payment is processed the room will be booked and show a confirmation page.
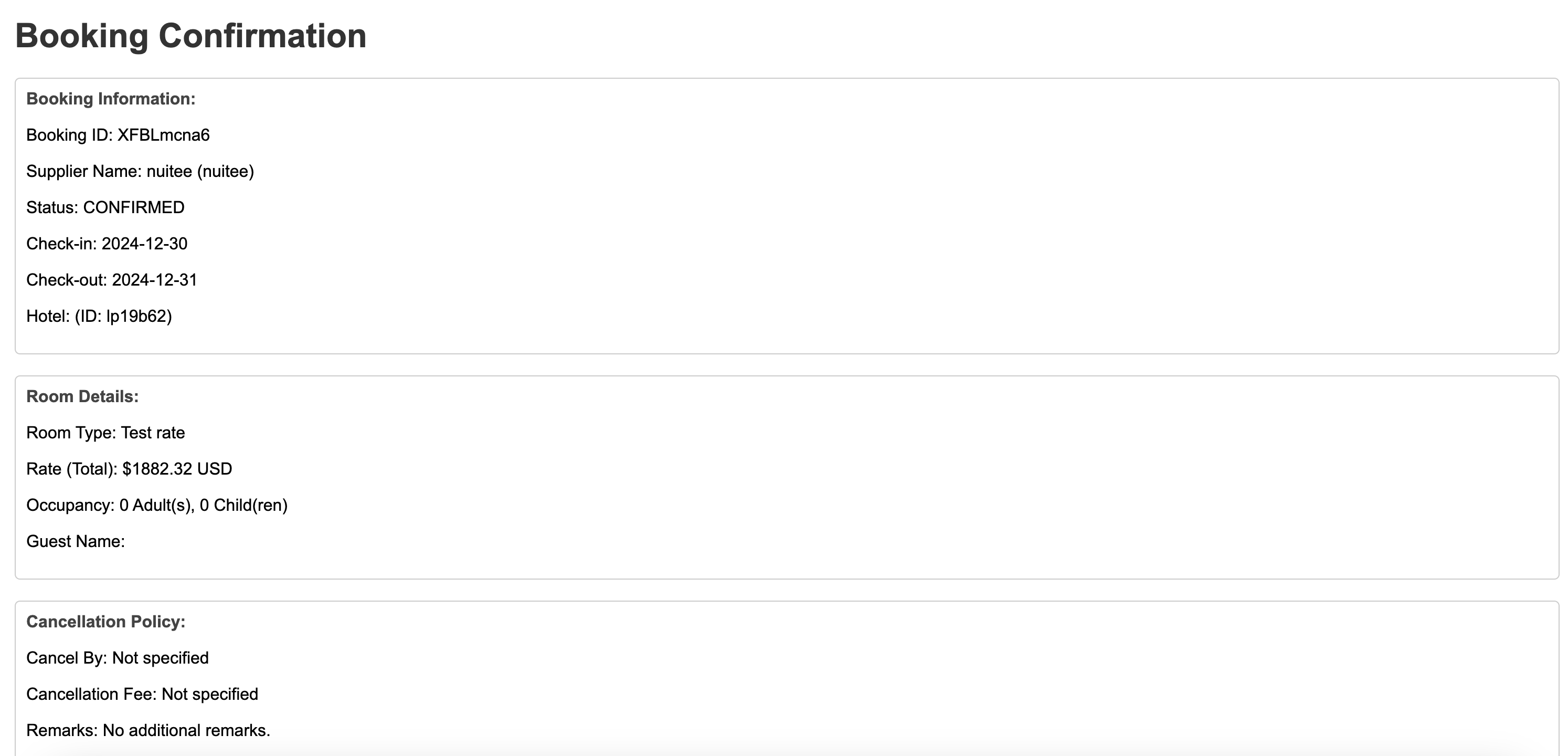
Step 9: Viewing the Booking in the Dashboard
The bookings will show in the dashboard of the API key you are using. The toggle at the top determines if the bookings and reports you see are from the sandbox or production key.
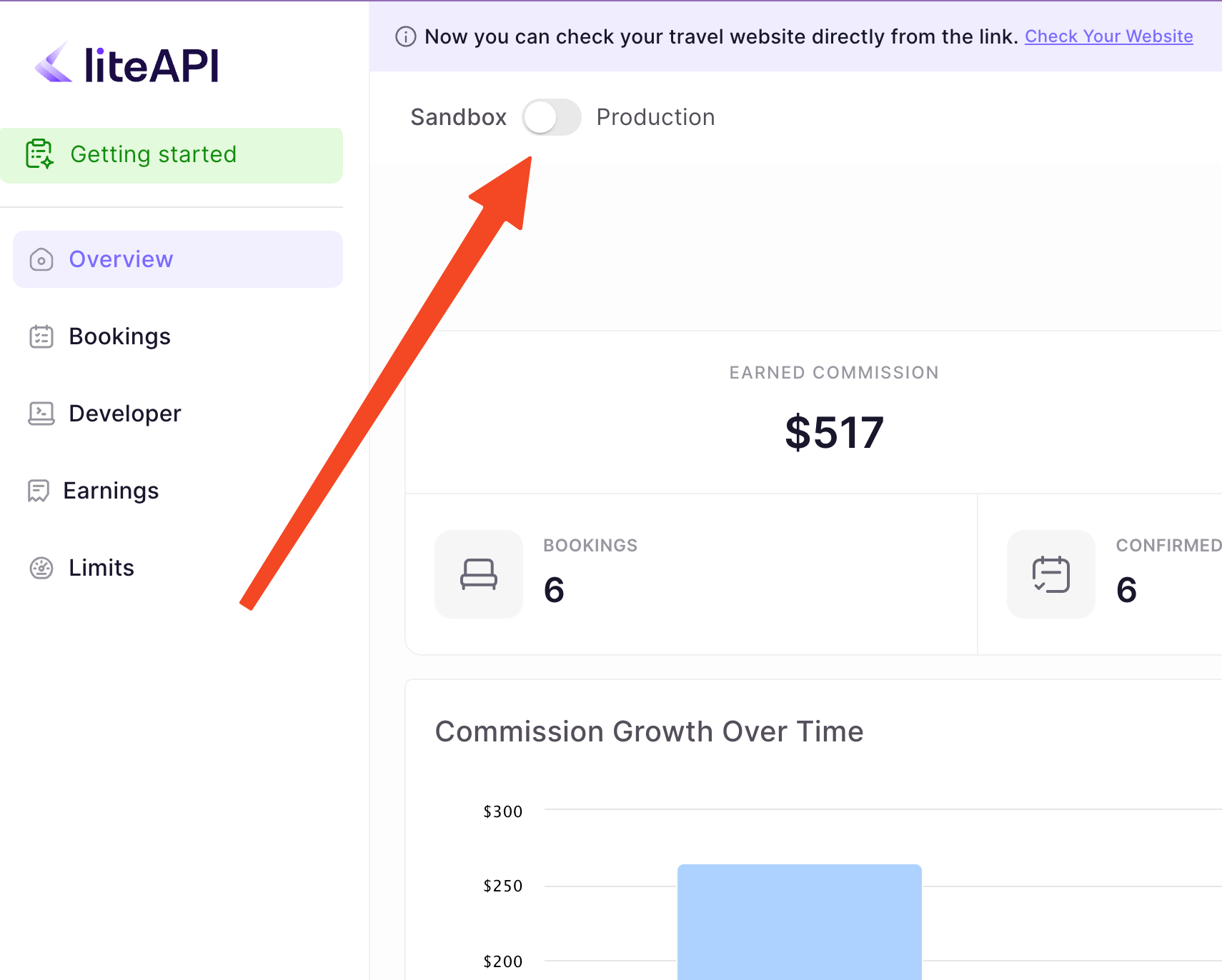
On the booking page you will see the new booking listed.

On the main dashboard you will see bookings reflected in the reports. Start by making a few sandbox bookings to see how your reports change.
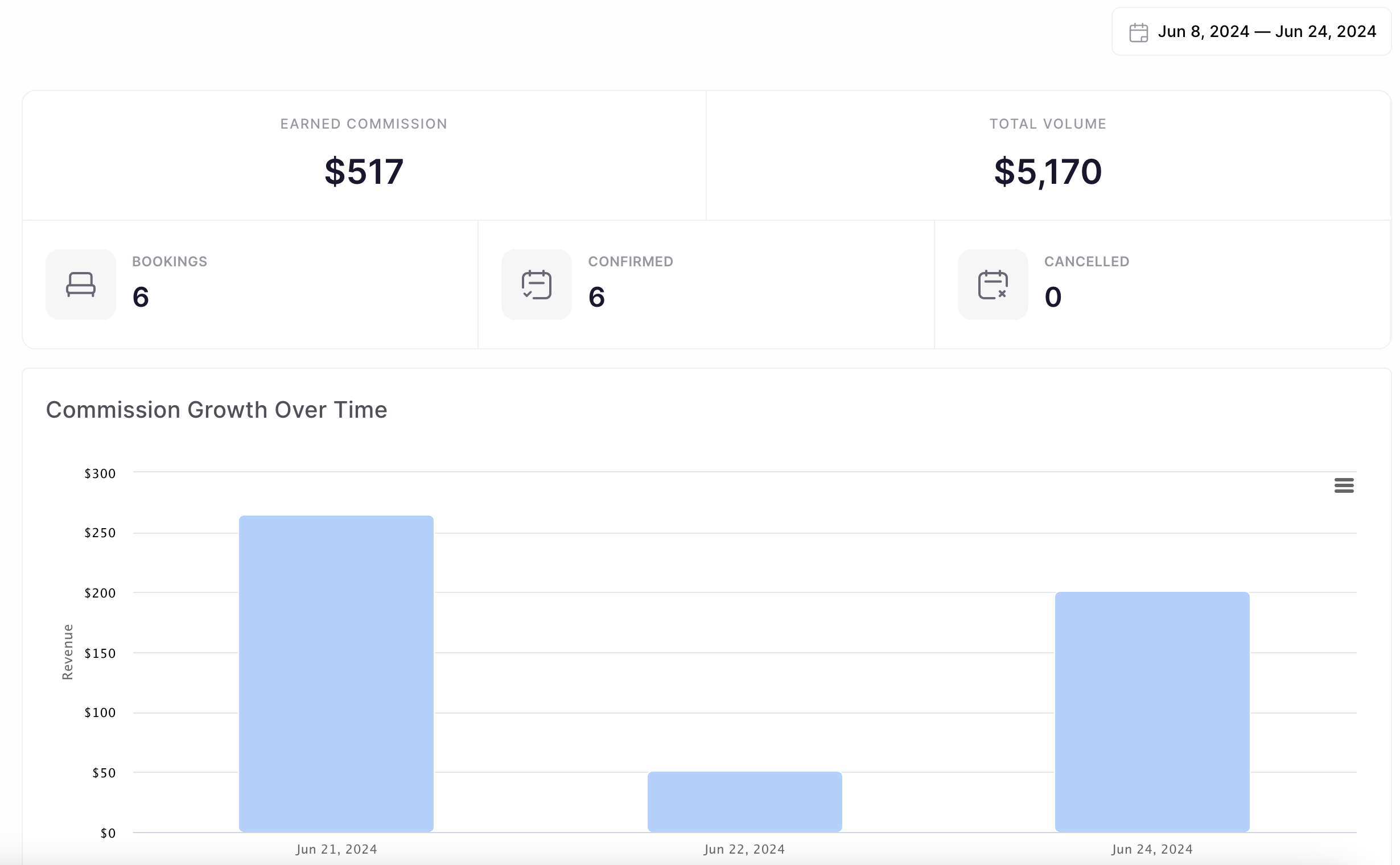
Conclusion
You now have a basic example app running on a Node.js server using Express. This app allows you to search for rates, pay via the liteAPI payment SDK, and book a room. Feel free to start fresh or clone the code and start customizing to build your own travel application with liteAPI.
Updated 17 days ago