Room Details
Room details can be accessed by enabling an optional flag in the rate query and combining the returned data with the hotel’s information. If your goal is to display room-specific details, this guide will help. A rate isn’t just about the price—it includes important details about the hotel and the room, such as its size and amenities, which are crucial for guests. This is where room mapping becomes essential. With room mapping, you can associate a specific rate with a particular room within the hotel. This allows you to display detailed room information alongside the rate, making it clear to guests what features and benefits come with their selection. To start, when querying for rates set roomMapping: true
like so:
const options = {
method: 'POST',
headers: {
accept: 'application/json',
'content-type': 'application/json',
'X-API-Key': 'sand_c0155ab8-c683-4f26-8f94-b5e92c5797b9'
},
body: JSON.stringify({
hotelIds: ['lp3803c'],
occupancies: [{adults: 1}],
currency: 'USD',
guestNationality: 'US',
checkin: '2025-12-30',
checkout: '2025-12-31',
roomMapping: true
})
};
fetch('https://api.liteapi.travel/v3.0/hotels/rates', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
This will return a mappedRoomId
as part of the rate offering if the hotel is mapped in liteAPI. This mappedRoomId
that can be used to locate that specific room's photos and information by locating the rooms
object with that id
.
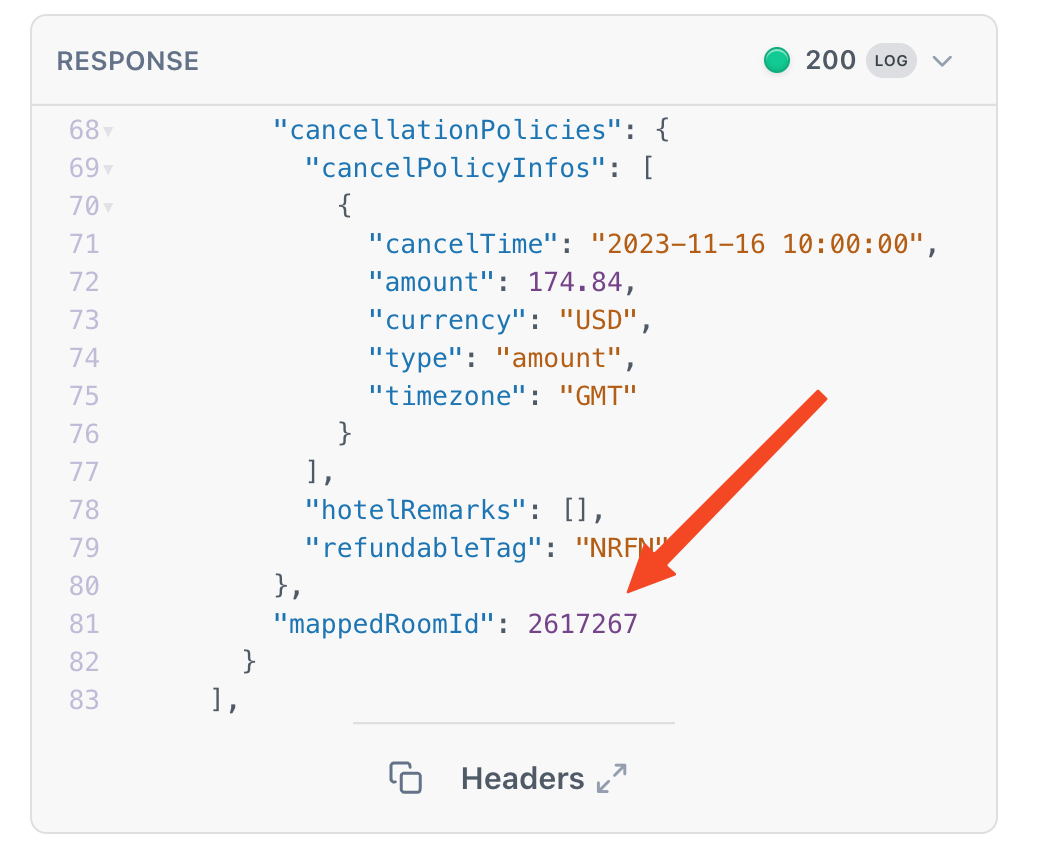
To display room details, iterate over the rooms
array and extract relevant information from each room object like:
roomName
-- ⭐️ NOTE: Always show the supplier room name as it can include important information, and is what will be sent to the hotel. This name is for reference purposes only.description
maxOccupancy
bedTypes
roomAmenities
In addition, to display the images, iterate over the room's photos
array and use the url
field.
Example:
"data": {
...
"rooms": [
{
"id": 5787126,
"roomName": "Studio King",
"description": "This studio includes an attached bathroom and a flat-screen TV and private balcony. The kitchenette features a stove, microwave and refrigerator.",
"roomSizeSquare": 33,
"roomSizeUnit": "m2",
"hotelId": "57871",
"maxAdults": 2,
"maxChildren": 2,
"maxOccupancy": 3,
"bedTypes": [
{
"quantity": 1,
"bedType": "Extra-large double bed(s) (Super-king size)",
"bedSize": "181-210 cm wide"
}
],
"roomAmenities": [
{
"amenitiesId": 9,
"name": "Telephone",
"sort": 113
}
],
"photos": [
{
"url": "https://snaphotelapi.com/rooms-large-pictures/322367522.jpg",
"imageDescription": "",
"imageClass1": "hotel room",
"imageClass2": "",
"failoverPhoto": "https://q-xx.bstatic.com/xdata/images/hotel/max1200/322367522.jpg?k=a0a9f05828252228ab4a876fef3304616cc8260e776735d83322bebaa0e1fb45&o=",
"mainPhoto": false,
"score": 4.53,
"classId": 1,
"classOrder": 1
},
{
"url": "https://snaphotelapi.com/rooms-large-pictures/322367511.jpg",
"imageDescription": "",
"imageClass1": "hotel room",
"imageClass2": "",
"failoverPhoto": "https://q-xx.bstatic.com/xdata/images/hotel/max1200/322367511.jpg?k=9c3b9357535b353eff4e1a7ac0c5088c7d331dbc3405264f455921436210dadf&o=",
"mainPhoto": false,
"score": 4.55,
"classId": 1,
"classOrder": 1
},
...
]
},
...
],
...
}
<h2>Rooms</h2>
<div>
<h3>Studio King</h3>
<img width="350px" src="https://snaphotelapi.com/rooms-large-pictures/322367522.jpg" />
<img width="350px" src="https://snaphotelapi.com/rooms-large-pictures/322367511.jpg" />
<p>This studio includes an attached bathroom and a flat-screen TV and private balcony...</p>
<p>Max Occupancy: 3 people</p>
<p>Beds: 1 Extra-large double bed (Super-king size)</p>
<h4>Amenities</h4>
<ul>
<li>Telephone</li>
<!-- Add more amenities as needed -->
</ul>
<!-- Add more rooms as needed -->
</div>
Summary
Using room mapping, you can supply your guests with detailed information about the room they want to stay in. This level of detail can give guests confidence about the specifics of the room they are staying in.
Updated 14 days ago